Issue with SwiftUI List and Button in Popover
Recently, I had an issue where I’ve wanted to display a SwiftUI List and Button together in a Popover. The idea was to have a List of items and a button to clear the list.
My code was as follows:
import SwiftUI
struct ContentView: View {
@State private var showingPopover = false
var body: some View {
Text("Popover Test")
.toolbar {
ToolbarItem() {
Button(action: {showingPopover = true}) {
Image(systemName: "list.bullet.rectangle.portrait.fill")
}
.popover(isPresented: $showingPopover) {
PopoverView()
}
}
}
}
}
struct PopoverView: View {
@State var testData = ["one", "two", "three", "four"]
var body: some View {
VStack() {
Button(action: {}) {
Text("Button 1")
}
.padding(10)
List(testData, id: \.self) { item in
Text(item)
}
}
}
}
However, this didn’t work in the way I’d expected. Only the Button displayed in the Popover.
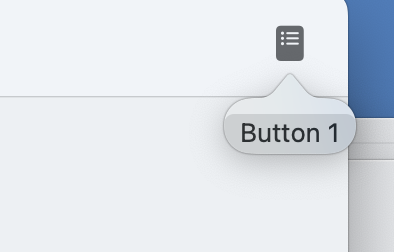
If I commented out the Button code, the List displayed, as expected.
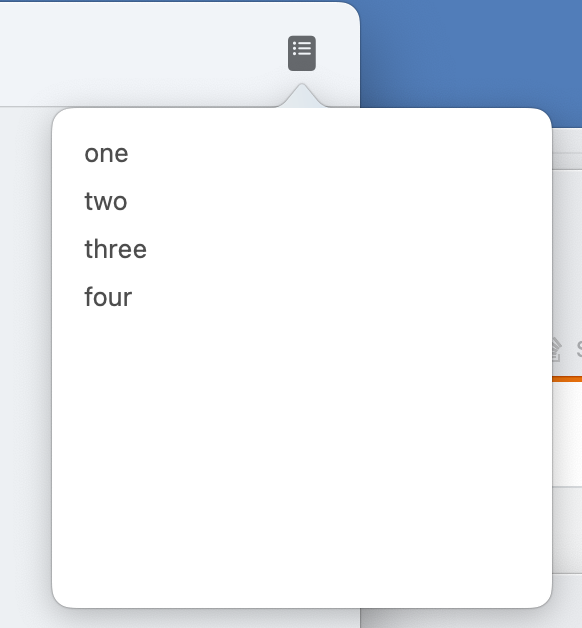
It took a while to get to the bottom if this problem, with the help of StackOverflow. Adding a .frame to the VStack fixed the issue.
VStack() {
Button(action: {}) {
Text("Button 1")
}
.padding(10)
List(testData, id: \.self) { item in
Text(item)
}
}
// Adding this next line fixes the issue
.frame(width: 222, height: 200)
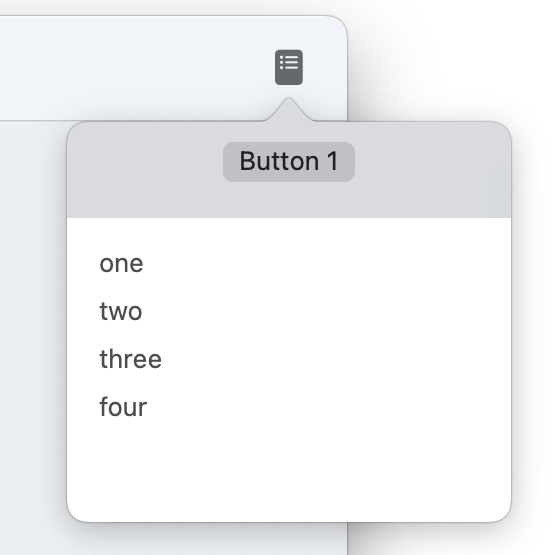
The problem occurs with Xcode 15.2 in MacOS 13 & 14 (tested to 14.2) and iPadOS 17.1.
The Code is on Github: https://github.com/tevendale/ListButtonPopover.